posix_spawnp hangs until child process terminates on Debian 9Debian 6 xsession hangs after loginDropbear...
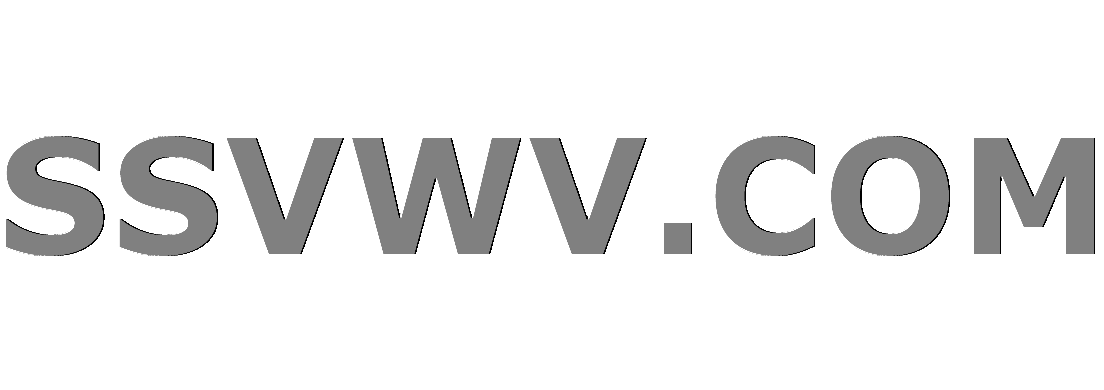
Multi tool use
Names of the Six Tastes
Using SUBSTRING and RTRIM Together
Not taking the bishop by the knight, why?
What can cause an unfrozen indoor copper drain pipe to crack?
Using wilcox.test() and t.test() in R yielding different p-values
Company stopped paying my salary. What are my options?
Lorentz invariance of Maxwell's equations in matter
Two (probably) equal real numbers which are not proved to be equal?
Is there a need for better software for writers?
Gift for mentor after his thesis defense?
What dice to use in a game that revolves around triangles?
Is it a Munchausen Number?
Are on’yomi words loanwords?
Can I bring back Planetary Romance as a genre?
Which spells are in some way related to shadows or the Shadowfell?
Rusty Chain and back cassette – Replace or Repair?
A Latin text with dependency tree
Compactness in normed vector spaces.
Row vectors and column vectors (Mathematica vs Matlab)
TeX Gyre Pagella Math Integral sign much too small
Locked my sa user out
How to avoid making self and former employee look bad when reporting on fixing former employee's work?
Was Mohammed the most popular first name for boys born in Berlin in 2018?
Has there been evidence of any other gods?
posix_spawnp hangs until child process terminates on Debian 9
Debian 6 xsession hangs after loginDropbear terminates before LUKS password prompt on Debian JessieDebian hangs when i'm inserting TLWN722NDebian Stretch hangs on rebootupdate-initramfs hangs on debian StretchWhen I log in, it hangs until crng init doneAre containers transparent to POSIX parent-child process relationships?Debian stretch: Kernel panic hangs Gnome desktopIs it safe to close a directory stream (DIR *) in both the parent and child process?Debian xarchiver “Failed to execute child process ”ar“ (No such file or directory)”
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ margin-bottom:0;
}
We recently found an interesting case where posix_spawnp hangs until the child process it spawned terminates on Debian 9. This is not reproducible on other distros like Ubuntu(18.04) or CentOS(7.3). You could use the snippet at the end to reproduce it. Just compile it and run ./test_posix_spawnp sleep 30
, assuming you named the executable as test_posix_spawnp. We passed in sleep 30
just to keep the child process running for a short while. You would see PID of child: xxx
is not printed immediately as an indicator.
The sample code below is to mock our real code, the key of which is to close all the file descriptors in the child process except stdin/stdout/stderr and the one that opened for logging and redirect stdout/stderr to the logging file. In either the real case or this mocking one, it seems that the child process has been spawned and it has started running the executable being passed.
Our questions:
Has anyone came across this issue before? Does it sound like a libc(2.24) bug? If not, how can we fix our code? If so, what are we supposed to do?
P.S. not sure if it matters but we observed that when it's reproducible or when on Debian, there is an extra pipe created during the execution of posix_spawnp, the parent has the read end and the child the write end.
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <spawn.h>
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
#include <wait.h>
#include <errno.h>
#define errExit(msg) do { perror(msg);
exit(EXIT_FAILURE); } while (0)
#define errExitEN(en, msg)
do { errno = en; perror(msg);
exit(EXIT_FAILURE); } while (0)
char **environ;
int
main(int argc, char *argv[])
{
pid_t child_pid;
int s, status;
sigset_t mask;
posix_spawnattr_t attr;
posix_spawnattr_t *attrp;
posix_spawn_file_actions_t file_actions;
posix_spawn_file_actions_t *file_actionsp;
attrp = NULL;
file_actionsp = NULL;
long open_max = sysconf(_SC_OPEN_MAX);
printf("sysconf says: max open file descriptor %ldn", open_max);
if (open_max > 32768) {
open_max = 32768;
printf("bump max open file desriptor to %ldn", open_max);
}
int flags = O_WRONLY | O_CREAT | O_APPEND;
mode_t mode = S_IRUSR | S_IWUSR | S_IRGRP | S_IRGRP;
int log_fd = open("test_posix_spawnp.log", flags, mode);
printf("opened output file "test_posix_spawnp.log", fd=%dn", log_fd);
/* Close all fds except log_fd to which stdout and stderr are redirected */
s = posix_spawn_file_actions_init(&file_actions);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_init");
s = posix_spawn_file_actions_adddup2(&file_actions, log_fd, STDOUT_FILENO);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_adddup2");
s = posix_spawn_file_actions_adddup2(&file_actions, log_fd, STDERR_FILENO);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_adddup2");
for (int i = 3; i < open_max; ++i) {
if (i == log_fd) continue;
s = posix_spawn_file_actions_addclose(&file_actions, i);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_addclose");
}
file_actionsp = &file_actions;
s = posix_spawnp(&child_pid, argv[optind], file_actionsp, attrp,
&argv[optind], environ);
if (s != 0)
errExitEN(s, "posix_spawn");
printf("PID of child: %ldn", (long) child_pid);
/* Clean up after ourselves */
if (file_actionsp != NULL) {
s = posix_spawn_file_actions_destroy(file_actionsp);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_destroy");
}
exit(EXIT_SUCCESS);
}
debian posix
New contributor
Johnny is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
We recently found an interesting case where posix_spawnp hangs until the child process it spawned terminates on Debian 9. This is not reproducible on other distros like Ubuntu(18.04) or CentOS(7.3). You could use the snippet at the end to reproduce it. Just compile it and run ./test_posix_spawnp sleep 30
, assuming you named the executable as test_posix_spawnp. We passed in sleep 30
just to keep the child process running for a short while. You would see PID of child: xxx
is not printed immediately as an indicator.
The sample code below is to mock our real code, the key of which is to close all the file descriptors in the child process except stdin/stdout/stderr and the one that opened for logging and redirect stdout/stderr to the logging file. In either the real case or this mocking one, it seems that the child process has been spawned and it has started running the executable being passed.
Our questions:
Has anyone came across this issue before? Does it sound like a libc(2.24) bug? If not, how can we fix our code? If so, what are we supposed to do?
P.S. not sure if it matters but we observed that when it's reproducible or when on Debian, there is an extra pipe created during the execution of posix_spawnp, the parent has the read end and the child the write end.
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <spawn.h>
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
#include <wait.h>
#include <errno.h>
#define errExit(msg) do { perror(msg);
exit(EXIT_FAILURE); } while (0)
#define errExitEN(en, msg)
do { errno = en; perror(msg);
exit(EXIT_FAILURE); } while (0)
char **environ;
int
main(int argc, char *argv[])
{
pid_t child_pid;
int s, status;
sigset_t mask;
posix_spawnattr_t attr;
posix_spawnattr_t *attrp;
posix_spawn_file_actions_t file_actions;
posix_spawn_file_actions_t *file_actionsp;
attrp = NULL;
file_actionsp = NULL;
long open_max = sysconf(_SC_OPEN_MAX);
printf("sysconf says: max open file descriptor %ldn", open_max);
if (open_max > 32768) {
open_max = 32768;
printf("bump max open file desriptor to %ldn", open_max);
}
int flags = O_WRONLY | O_CREAT | O_APPEND;
mode_t mode = S_IRUSR | S_IWUSR | S_IRGRP | S_IRGRP;
int log_fd = open("test_posix_spawnp.log", flags, mode);
printf("opened output file "test_posix_spawnp.log", fd=%dn", log_fd);
/* Close all fds except log_fd to which stdout and stderr are redirected */
s = posix_spawn_file_actions_init(&file_actions);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_init");
s = posix_spawn_file_actions_adddup2(&file_actions, log_fd, STDOUT_FILENO);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_adddup2");
s = posix_spawn_file_actions_adddup2(&file_actions, log_fd, STDERR_FILENO);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_adddup2");
for (int i = 3; i < open_max; ++i) {
if (i == log_fd) continue;
s = posix_spawn_file_actions_addclose(&file_actions, i);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_addclose");
}
file_actionsp = &file_actions;
s = posix_spawnp(&child_pid, argv[optind], file_actionsp, attrp,
&argv[optind], environ);
if (s != 0)
errExitEN(s, "posix_spawn");
printf("PID of child: %ldn", (long) child_pid);
/* Clean up after ourselves */
if (file_actionsp != NULL) {
s = posix_spawn_file_actions_destroy(file_actionsp);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_destroy");
}
exit(EXIT_SUCCESS);
}
debian posix
New contributor
Johnny is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
We recently found an interesting case where posix_spawnp hangs until the child process it spawned terminates on Debian 9. This is not reproducible on other distros like Ubuntu(18.04) or CentOS(7.3). You could use the snippet at the end to reproduce it. Just compile it and run ./test_posix_spawnp sleep 30
, assuming you named the executable as test_posix_spawnp. We passed in sleep 30
just to keep the child process running for a short while. You would see PID of child: xxx
is not printed immediately as an indicator.
The sample code below is to mock our real code, the key of which is to close all the file descriptors in the child process except stdin/stdout/stderr and the one that opened for logging and redirect stdout/stderr to the logging file. In either the real case or this mocking one, it seems that the child process has been spawned and it has started running the executable being passed.
Our questions:
Has anyone came across this issue before? Does it sound like a libc(2.24) bug? If not, how can we fix our code? If so, what are we supposed to do?
P.S. not sure if it matters but we observed that when it's reproducible or when on Debian, there is an extra pipe created during the execution of posix_spawnp, the parent has the read end and the child the write end.
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <spawn.h>
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
#include <wait.h>
#include <errno.h>
#define errExit(msg) do { perror(msg);
exit(EXIT_FAILURE); } while (0)
#define errExitEN(en, msg)
do { errno = en; perror(msg);
exit(EXIT_FAILURE); } while (0)
char **environ;
int
main(int argc, char *argv[])
{
pid_t child_pid;
int s, status;
sigset_t mask;
posix_spawnattr_t attr;
posix_spawnattr_t *attrp;
posix_spawn_file_actions_t file_actions;
posix_spawn_file_actions_t *file_actionsp;
attrp = NULL;
file_actionsp = NULL;
long open_max = sysconf(_SC_OPEN_MAX);
printf("sysconf says: max open file descriptor %ldn", open_max);
if (open_max > 32768) {
open_max = 32768;
printf("bump max open file desriptor to %ldn", open_max);
}
int flags = O_WRONLY | O_CREAT | O_APPEND;
mode_t mode = S_IRUSR | S_IWUSR | S_IRGRP | S_IRGRP;
int log_fd = open("test_posix_spawnp.log", flags, mode);
printf("opened output file "test_posix_spawnp.log", fd=%dn", log_fd);
/* Close all fds except log_fd to which stdout and stderr are redirected */
s = posix_spawn_file_actions_init(&file_actions);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_init");
s = posix_spawn_file_actions_adddup2(&file_actions, log_fd, STDOUT_FILENO);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_adddup2");
s = posix_spawn_file_actions_adddup2(&file_actions, log_fd, STDERR_FILENO);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_adddup2");
for (int i = 3; i < open_max; ++i) {
if (i == log_fd) continue;
s = posix_spawn_file_actions_addclose(&file_actions, i);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_addclose");
}
file_actionsp = &file_actions;
s = posix_spawnp(&child_pid, argv[optind], file_actionsp, attrp,
&argv[optind], environ);
if (s != 0)
errExitEN(s, "posix_spawn");
printf("PID of child: %ldn", (long) child_pid);
/* Clean up after ourselves */
if (file_actionsp != NULL) {
s = posix_spawn_file_actions_destroy(file_actionsp);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_destroy");
}
exit(EXIT_SUCCESS);
}
debian posix
New contributor
Johnny is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
We recently found an interesting case where posix_spawnp hangs until the child process it spawned terminates on Debian 9. This is not reproducible on other distros like Ubuntu(18.04) or CentOS(7.3). You could use the snippet at the end to reproduce it. Just compile it and run ./test_posix_spawnp sleep 30
, assuming you named the executable as test_posix_spawnp. We passed in sleep 30
just to keep the child process running for a short while. You would see PID of child: xxx
is not printed immediately as an indicator.
The sample code below is to mock our real code, the key of which is to close all the file descriptors in the child process except stdin/stdout/stderr and the one that opened for logging and redirect stdout/stderr to the logging file. In either the real case or this mocking one, it seems that the child process has been spawned and it has started running the executable being passed.
Our questions:
Has anyone came across this issue before? Does it sound like a libc(2.24) bug? If not, how can we fix our code? If so, what are we supposed to do?
P.S. not sure if it matters but we observed that when it's reproducible or when on Debian, there is an extra pipe created during the execution of posix_spawnp, the parent has the read end and the child the write end.
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <spawn.h>
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <string.h>
#include <wait.h>
#include <errno.h>
#define errExit(msg) do { perror(msg);
exit(EXIT_FAILURE); } while (0)
#define errExitEN(en, msg)
do { errno = en; perror(msg);
exit(EXIT_FAILURE); } while (0)
char **environ;
int
main(int argc, char *argv[])
{
pid_t child_pid;
int s, status;
sigset_t mask;
posix_spawnattr_t attr;
posix_spawnattr_t *attrp;
posix_spawn_file_actions_t file_actions;
posix_spawn_file_actions_t *file_actionsp;
attrp = NULL;
file_actionsp = NULL;
long open_max = sysconf(_SC_OPEN_MAX);
printf("sysconf says: max open file descriptor %ldn", open_max);
if (open_max > 32768) {
open_max = 32768;
printf("bump max open file desriptor to %ldn", open_max);
}
int flags = O_WRONLY | O_CREAT | O_APPEND;
mode_t mode = S_IRUSR | S_IWUSR | S_IRGRP | S_IRGRP;
int log_fd = open("test_posix_spawnp.log", flags, mode);
printf("opened output file "test_posix_spawnp.log", fd=%dn", log_fd);
/* Close all fds except log_fd to which stdout and stderr are redirected */
s = posix_spawn_file_actions_init(&file_actions);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_init");
s = posix_spawn_file_actions_adddup2(&file_actions, log_fd, STDOUT_FILENO);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_adddup2");
s = posix_spawn_file_actions_adddup2(&file_actions, log_fd, STDERR_FILENO);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_adddup2");
for (int i = 3; i < open_max; ++i) {
if (i == log_fd) continue;
s = posix_spawn_file_actions_addclose(&file_actions, i);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_addclose");
}
file_actionsp = &file_actions;
s = posix_spawnp(&child_pid, argv[optind], file_actionsp, attrp,
&argv[optind], environ);
if (s != 0)
errExitEN(s, "posix_spawn");
printf("PID of child: %ldn", (long) child_pid);
/* Clean up after ourselves */
if (file_actionsp != NULL) {
s = posix_spawn_file_actions_destroy(file_actionsp);
if (s != 0)
errExitEN(s, "posix_spawn_file_actions_destroy");
}
exit(EXIT_SUCCESS);
}
debian posix
debian posix
New contributor
Johnny is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Johnny is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Johnny is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 1 hour ago
JohnnyJohnny
11
11
New contributor
Johnny is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Johnny is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "106"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Johnny is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f517894%2fposix-spawnp-hangs-until-child-process-terminates-on-debian-9%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Johnny is a new contributor. Be nice, and check out our Code of Conduct.
Johnny is a new contributor. Be nice, and check out our Code of Conduct.
Johnny is a new contributor. Be nice, and check out our Code of Conduct.
Johnny is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Unix & Linux Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f517894%2fposix-spawnp-hangs-until-child-process-terminates-on-debian-9%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qQgsXkbx7RE